Wednesday, 2 February 2011
I was cleaning out some miscellaneous files from my computer today when I found a folder called “eyeos”. EyeOS. My friend and I thought we were uber-cool when we set up eyeOS on our shared hosting accounts and looking back, yeah, well, we were.
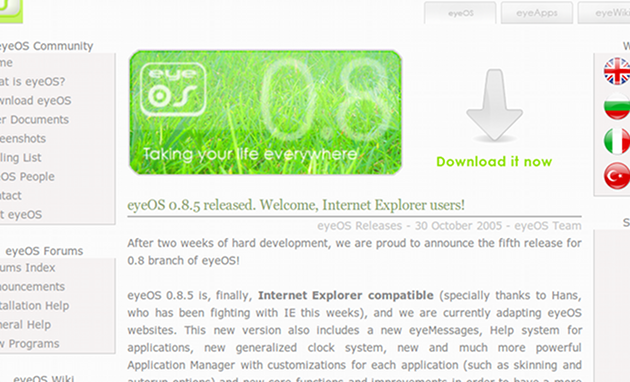
eyeOS.org, ca. November, 2005. Very Web 1.9.
EyeOS was an ambitious open-source project to give you a desktop in your browser. You could have files, record appointments, and write documents from anywhere, as long as you had an Internet-connected computer. Nothing was stored on your local hard drive, it was all up on the … Internet.
This was November, 2005. This was back when clouds meant fewer UV rays.
(more…)
Posted in Internets | No Comments »
Monday, 10 January 2011
One of the biggest hurdles in going from JavaScript as a trivial gimmick for making divs change color to a powerful language for building real applications is its single-namespace weakness. It’s easy to accidentally cause variable names to collide when you start combining scripts, and there’s no native library or module system to alleviate this. For example, jQuery, prototype.js, and MooTools all want to use “$” as a shortcut variable, which has caused me problems before when combining software written by other people.
It’s easy to write a bunch of JavaScript that drops variables all over the global namespace, but often, you want to reuse some JavaScript component in multiple pages. Let’s look at a hypothetical example:
(more…)
Posted in Internets, Programming | No Comments »
Monday, 6 December 2010
I’ve been looking around for Scheme interpreters written in JavaScript for a while now. The original idea was to make some kind of interactive online teaching tool, like an interactive version of The Little Schemer.
Googling for “javascript scheme” turns up a few implementations:
(more…)
Posted in Programming | No Comments »
Friday, 19 November 2010
Templating is one of those must-haves for building websites; separating content from presentation is sliced bread compared to cavalierly interleave logic and HTML into one file. In Scheme, I think that quasiquotation could be used in wonderful ways to create templates; namely, every template can be a function that simply takes parameters and directly uses quasiquotation as the templater:
[clojure]
(define blog-post-template
(lambda (title date message comments)
`(html
(head (title "My Blog – " ,title))
(body
(div (@ (class "post"))
(h1 ,title)
,message)
(div (@ (class "comments"))
(p ,comments) …)))))
[/clojure]
and then to use it, you would just use
[clojure]
(blog-post-template
"Another pedantic blog post"
"Nov 20, 2010"
"Today I’m going to complain about everything …"
‘("Awesome blog!"
"Actually, I disagree with you on everything"
"Spam"))
[/clojure]
You could also add a little more flexibility into your template by accepting an association list of parameters rather than fixed parameters:
[clojure]
(define blog-post-template
(lambda params
`(html
(head (title "My Blog – " ,(cadr (assq ‘title params))))
(body))))
[/clojure]
which can be made easier to use by redefining quasiquote with some templating-specific conveniences.
Posted in Internets, Programming | No Comments »
Tuesday, 16 November 2010
An Ubuntu package of Petite Chez Scheme seems to be a common request among students, given the popularity of Ubuntu among the techno-capable. Currently, only RPM packages are provided on the Chez Scheme site, and the traditional recommendation was to use alien to convert the RPM into a .deb. There’s no reason to have everybody do this though.
I’ve built a .deb package of Petite Chez Scheme for amd64 (64-bit) Ubuntu 10.10. You should be able to install it by simply double-clicking the package and following the on-screen prompts. Petite can then be run using the terminal command `petite`.
Known issues: No package docs.
Posted in Linux, Programming | 2 Comments »
Friday, 15 January 2010
Update: I now have an improved C version of this program: usbscale.
I got suckered into one of those hard-to-cancel Stamps.com trials. The upside is that they give you a $10 USB 5 lb. scale to use with their software. The downside is that they want you to only use it with their software, and the company that makes the scale has since taken down their free USB-scale program.
The good news, as Nicholas Piasecki and some Linux users figured out, is that the USB scale conforms to the USB HID specifications, which helpfully standardize how USB scales should work (no joke).
So, this little Perl hack reads from the Stamps.com scale by accessing the hidraw#
interface that Linux provides. In my case, I have hidraw4
hard-coded into the script itself. Basically, it loops until it reads a good value from the scale, at which point it prints out the weight and exits.
Edit: This code is now a Gist on GitHub.
(more…)
Posted in Hardware, Linux, Programming, Standards | 11 Comments »
Monday, 20 April 2009
The symptom is typical: you check out the partitions on your hard drive in Windows Disk Manager only to find out that there’s a weird, inaccessible partition that’s of the type “EISA Configuration.” What is it? Can I get rid of it?
What is it?
It has become standard practice for manufacturers to include recovery data or utilities on the hard drive to save them the costs of creating separate recovery disks for your computer. The benefit is that you can always restore your computer without worrying about losing your restory disks, but the downside is that it’s taking space on your hard drive, and if your hard drive died, you don’t have any restore disks at all.
(more…)
Posted in Uncategorized | 3 Comments »
Thursday, 2 April 2009
Objective: Create a single-file PHP installer for WordPress that will automate the downloading, unpacking, and setup of a WordPress blog.
Reason: As of right now, setting up a WordPress blog involves a lot of fiddling with files. The user must download the archive, unzip it on their computer, open a ftp connection to their server, upload all of the contents, hope that all the permissions are right, and navigate to the right directory. Since WordPress is such a popular blogging software, many people (most of whom are not familiar with a Unix shell or do not have access to one) would benefit from a single-file installer.
Deliverable: One (1) PHP file. No other files can be included with this (unless embedded), since this would void much of the advantage of such a single-file installer.
Basic procedure (a rough plan):
- Instruct the user on the requirements of WordPress (needs hosting with PHP, etc.).
- Instruct the user on downloading and uploading this installer, and subsequently running it.
- Check the server environment for required components, correct permissions, etc.
- Give the user some options on name, location, etc. and solicit other options like database credentials.
- Download the latest WordPress distribution.
- Unpack the distribution to the right place.
- Proceed more-or-less with the “usual” install.
Posted in Internets, Programming | No Comments »
Tuesday, 3 March 2009
Firefox Quick Searches have become an ingrained habit for me after a lot of repeat searching. Recently I’ve been working on a Java project and I’ve found myself needing to look up classes in the Java online documentation quite often. To that end, I’ve set up a quick-search bookmark that will take me to the relevant documentation page by typing java [keyword]
in the address bar. It uses Google’s I’m Feeling Lucky search:

Just add a bookmark with the string “http://www.google.com/search?btnI=I%27m+Feeling+Lucky&ie=UTF-8&oe=UTF-8&q=Java%206%20%s
“.
Posted in Uncategorized | No Comments »
Saturday, 10 January 2009
Update: There used to be an unhappy blog post about my experience with Musicnotes.com from 2009 here, but I don’t know if any of it is relevant or not anymore. If you are looking for open-source software for music notation, I encourage you to check out MuseScore.
Posted in Internets | 42 Comments »